Statiq Framework is a powerful static generation framework that can be used to create custom static generation applications. While many users will find that Statiq Web or Statiq Docs have enough functionality built-in, crafting a custom static generator with Statiq Framework provides the most flexibility.
Quick Start
The easiest way to get started with Statiq Framework is to install the Statiq.App package into a .NET Core console application and use the bootstrapper to configure everything.
There's no statiq.exe
. Unlike other static generators which ship as a self-contained executable, Statiq is a framework and as such it runs from within your own console application. This provides the greatest flexibility and extensibility and is one of the unique aspects of using Statiq.
Step 1: Install .NET Core
Statiq Framework consists of .NET Core libraries and installing the .NET Core SDK is the only prerequisite.
Step 2: Create a .NET Core Console Application
Create a new console application using the dotnet
command-line interface:
dotnet new console -o MyGenerator
Step 3: Install Statiq.App
dotnet add package Statiq.App --version x.y.z
Use whatever is the most recent version of Statiq.App. The --version
flag is needed while the package is pre-release.
Step 4: Create a Bootstrapper
There are several ways to create and configure an engine, but by far the easiest is to use the bootstrapper. Add the following code in your Program.cs
file:
using System;
using System.Threading.Tasks;
using Statiq.App;
namespace MyGenerator
{
public class Program
{
public static async Task<int> Main(string[] args) =>
await Bootstrapper
.Factory
.CreateDefault(args)
.RunAsync();
}
}
Alternatively if you're using .NET 6 and have <ImplicitUsings>enable</ImplicitUsings>
in your project file,
you can make use of top-level statements and implicit usings to simplify your Program.cs
file
and this is all that's needed:
return await Bootstrapper
.Factory
.CreateDefault(args)
.RunAsync();
This creates a default Bootstrapper
instance and passes it the command-line arguments so it can process them with the .CreateDefault(args)
call. Then it executes the specified command (from the command-line) during the final .RunAsync()
call.
This example is all you need for a minimal, functioning Statiq Framework application. The only problem is that it doesn’t actually do anything. Let’s add one more step and process some Markdown files.
Step 5: Add a Pipeline and Modules
Most functionality in Statiq Framework is provided by pipelines and modules. The bootstrapper has several mechanisms for adding and defining pipelines. For this last step lets add a quick pipeline to read Markdown files, render them, and write them back out to disk using some fluent methods to define a pipeline and add modules to it:
using System;
using System.Threading.Tasks;
using Statiq.App;
using Statiq.Markdown;
namespace MyGenerator
{
public class Program
{
public static async Task<int> Main(string[] args) =>
await Bootstrapper
.Factory
.CreateDefault(args)
.BuildPipeline("Render Markdown", builder => builder
.WithInputReadFiles("*.md")
.WithProcessModules(new RenderMarkdown())
.WithOutputWriteFiles(".html"))
.RunAsync();
}
}
While Markdown support is part of Statiq Framework (and covered by the same MIT license), you'll also need to add the Statiq.Markdown package to get access to the RenderMarkdown
module used in the above code:
dotnet add package Statiq.Markdown --version x.y.z
That's because Statiq Framework is completely general and doesn't make any assumptions about the kind of generator you're building (unlike Statiq Web which is somewhat opinionated and provides support for templates and more out of the box). Many other Statiq Framework extensions also exist and you should pick and choose which ones you need.
Step 6: Run it!
Let the magic happen:
dotnet run
Next Steps
📖 Read the guide to learn more about all the features of Statiq.
💬 Use the Discussions for assistance, questions, and general discussion about all Statiq projects.
🐞 File an issue if you find a bug or have a feature request related to Statiq Framework.
How It Works
Statiq is powerful because it combines a few simple building blocks that can be rearranged and used in limitless combinations. Think of it like LEGO® for static generation.
Content and data can come from a variety of sources.
Documents are created that each contain content or data and metadata.
The documents are processed by pipelines.
Each pipeline consists of one or more modules that manipulate the documents given to it by transforming, aggregating, filtering, or producing entirely new documents.
The final output of each pipeline is made available to other pipelines and may be written to output files or deployed to hosting services.
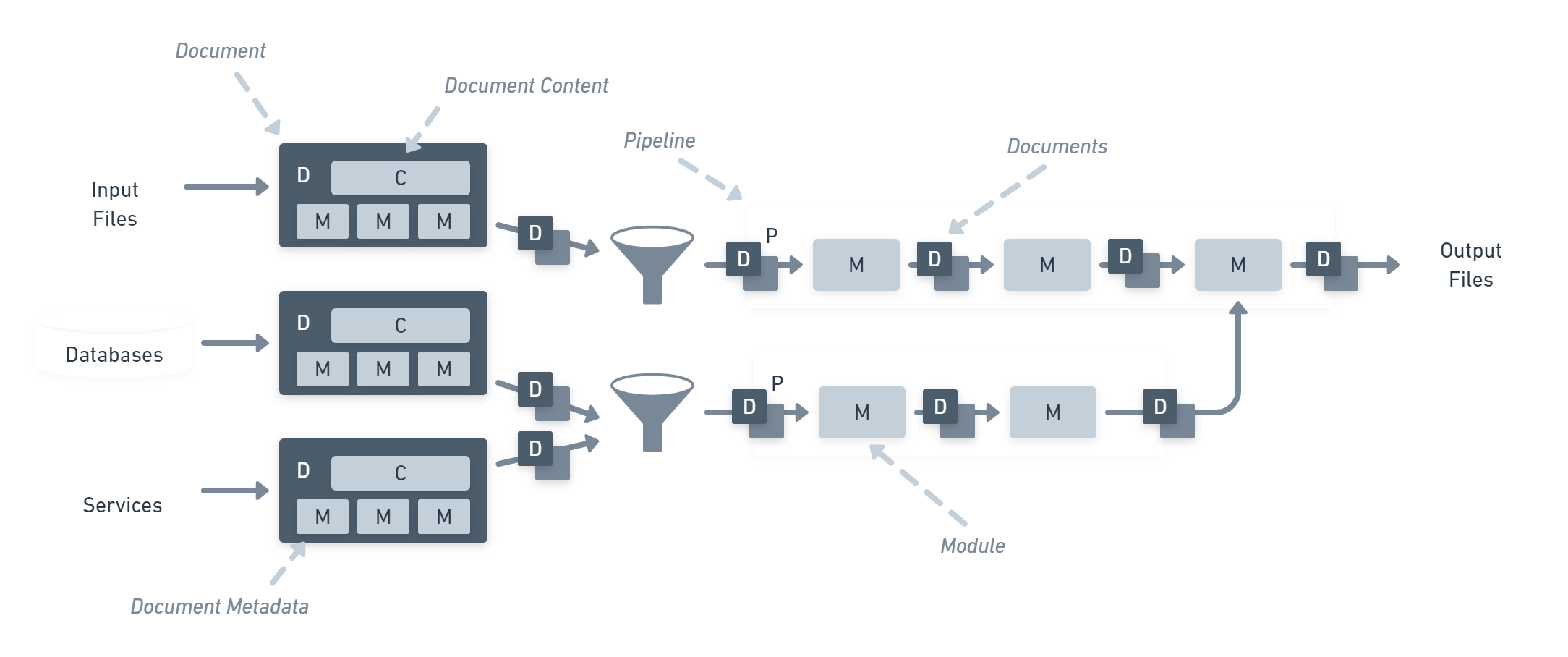